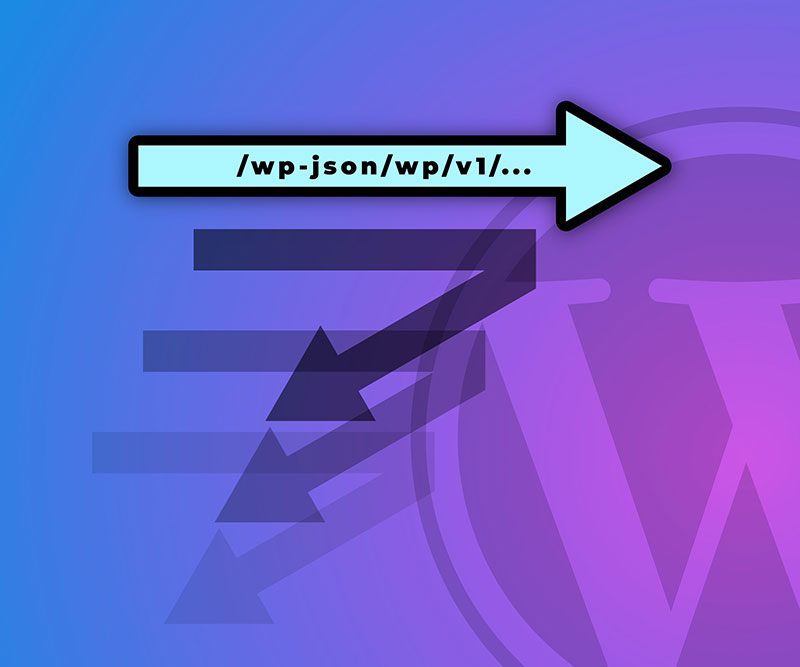
WordPress cung cấp nhiều hàm thuộc nhóm wp_send_json_*
để trả về dữ liệu JSON trong các ứng dụng AJAX hoặc REST API. Dưới đây là danh sách các hàm và mô tả chi tiết:
1. wp_send_json()
#
- Mô tả: Trả về dữ liệu JSON đã mã hóa và dừng script.
- Cách sử dụng:
wp_send_json( $data );
- Tham số:
$data
(mixed): Dữ liệu cần trả về (mảng, đối tượng, hoặc chuỗi). - Ví dụ:
$response = ['status' => 'success', 'message' => 'Data saved!']; wp_send_json( $response );
2. wp_send_json_success()
#
- Mô tả: Trả về dữ liệu JSON với trạng thái thành công (
success: true
). - Cách sử dụng:
wp_send_json_success( $data, $status_code );
- Tham số:
$data
(mixed): Dữ liệu cần trả về.$status_code
(int): Mã trạng thái HTTP (mặc định là 200).
- Ví dụ:
wp_send_json_success( ['message' => 'Operation completed!'] );
3. wp_send_json_error()
#
- Mô tả: Trả về dữ liệu JSON với trạng thái lỗi (
success: false
). - Cách sử dụng:
wp_send_json_error( $data, $status_code );
- Tham số:
$data
(mixed): Dữ liệu lỗi cần trả về.$status_code
(int): Mã trạng thái HTTP (mặc định là 500).
- Ví dụ:
wp_send_json_error( ['message' => 'An error occurred.'], 400 );
4. wp_send_json_data()
(deprecated)#
- Mô tả: Trả về dữ liệu JSON, tương tự
wp_send_json()
. Hàm này đã bị loại bỏ từ WordPress 5.5. - Cách sử dụng:
wp_send_json_data( $data );
So sánh các hàm wp_send_json_*
#
Hàm | Trạng thái | Dữ liệu trả về |
---|---|---|
wp_send_json() |
Tùy thuộc vào dữ liệu | Trả về JSON thô. |
wp_send_json_success() |
success: true | Trả về JSON trạng thái thành công. |
wp_send_json_error() |
success: false | Trả về JSON trạng thái lỗi. |
wp_send_json_data() |
Tùy thuộc vào dữ liệu | Tương tự wp_send_json() (deprecated). |
Khi nào sử dụng từng hàm?#
wp_send_json()
: Sử dụng khi bạn cần trả về JSON mà không cần trạng tháisuccess
.wp_send_json( ['custom_key' => 'custom_value'] );
wp_send_json_success()
: Sử dụng khi trả về dữ liệu và báo trạng thái thành công.wp_send_json_success( ['user_id' => 1] );
wp_send_json_error()
: Sử dụng khi có lỗi và muốn thông báo chi tiết lỗi.wp_send_json_error( ['error_code' => 'user_not_found'] );
Lưu ý khi sử dụng wp_send_json_*
:#
- Dừng script: Tất cả các hàm
wp_send_json_*
sẽ tự động dừng thực thi script (die()
hoặcexit()
). - HTTP Headers: Tự động thiết lập header
Content-Type: application/json
. - Ajax nonce: Nếu đang làm việc với AJAX trong WordPress, đừng quên kiểm tra nonce để bảo mật.
if ( ! check_ajax_referer( 'my_nonce', 'security', false ) ) { wp_send_json_error( ['message' => 'Invalid nonce'] ); }
Ví dụ:
Dưới đây là một ví dụ đơn giản minh họa cách sử dụng các hàmwp_send_json
, wp_send_json_success
, và wp_send_json_error
trong WordPress AJAX:
1. Tạo hàm xử lý AJAX#
Mở tệp PHP trong plugin hoặc tệpfunctions.php
của theme và thêm đoạn mã sau:
add_action('wp_ajax_my_ajax_action', 'handle_my_ajax_action');
add_action('wp_ajax_nopriv_my_ajax_action', 'handle_my_ajax_action');
function handle_my_ajax_action() {
// Kiểm tra nonce để bảo mật
if ( ! isset($_POST['security']) || ! wp_verify_nonce($_POST['security'], 'my_ajax_nonce') ) {
wp_send_json_error(['message' => 'Invalid nonce']);
}
// Lấy dữ liệu từ POST
$input = isset($_POST['input_data']) ? sanitize_text_field($_POST['input_data']) : '';
// Xử lý logic
if (empty($input)) {
wp_send_json_error(['message' => 'Input cannot be empty']);
} elseif ($input === 'test') {
wp_send_json_success(['message' => 'Test input received']);
} else {
wp_send_json(['custom_message' => 'Your input: ' . $input]);
}
}
2. JavaScript AJAX để gửi yêu cầu#
Thêm đoạn mã JavaScript này vào frontend (tệp JS hoặc inline script):jQuery(document).ready(function($) {
$('#ajaxForm').on('submit', function(e) {
e.preventDefault(); // Ngăn chặn submit form
const inputData = $('#inputField').val();
$.ajax({
url: ajaxurl, // WordPress cung cấp biến `ajaxurl` sẵn
type: 'POST',
dataType: 'json',
data: {
action: 'my_ajax_action', // Tên action
security: myAjax.security, // Nonce được cung cấp từ PHP
input_data: inputData
},
success: function(response) {
if (response.success) {
alert('Success: ' + response.data.message);
} else {
alert('Error: ' + response.data.message);
}
},
error: function() {
alert('AJAX request failed.');
}
});
});
});
3. Thêm Form HTML và Localize Script#
Thêm form vào tệp giao diện của bạn:<form id="ajaxForm">
<label for="inputField">Enter Data:</label>
<input type="text" id="inputField" name="input_data" />
<button type="submit">Submit</button>
</form>
<script>
var myAjax = {
security: "<?php echo wp_create_nonce('my_ajax_nonce'); ?>" // Tạo nonce
};
</script>
4. Kết quả khi gửi form#
Trường hợp 1: Input trống
- Output JSON:
{ "success": false, "data": { "message": "Input cannot be empty" } }
- Hiển thị trên alert:
Error: Input cannot be empty
Trường hợp 2: Input là test
- Output JSON:
{ "success": true, "data": { "message": "Test input received" } }
- Hiển thị trên alert:
Success: Test input received
Trường hợp 3: Input là chuỗi khác
- Output JSON:
{ "custom_message": "Your input: hello" }
- Hiển thị trên alert:
Success: Your input: hello
Tóm tắt:
wp_send_json_success
: Sử dụng khi cần trả về trạng thái thành công (success: true
).wp_send_json_error
: Sử dụng khi có lỗi hoặc yêu cầu không hợp lệ (success: false
).wp_send_json
: Tùy chỉnh dữ liệu trả về mà không quan tâm đến trạng thái (success
không được thiết lập).
Nội dung bài viết
- 1.
wp_send_json()
- 2.
wp_send_json_success()
- 3.
wp_send_json_error()
- 4.
wp_send_json_data()
(deprecated) - So sánh các hàm
wp_send_json_*
- Khi nào sử dụng từng hàm?
- Lưu ý khi sử dụng
wp_send_json_*
: - 1. Tạo hàm xử lý AJAX
- 2. JavaScript AJAX để gửi yêu cầu
- 3. Thêm Form HTML và Localize Script
- 4. Kết quả khi gửi form